Mono and Stereo Graphics
The Data Arena is built to primarily display stereoscopic graphics using an over/under system which targets the left and right eyes separately. This works by feeding a double-height image and merging duplicate imagery into two layers, alternating the visibility of each in sync with active 3D glasses. Slight differences in the position of elements between the two images allow for the perception of depth. With some simple modifications to your Processing sketches, you can take advantage of this system and add some depth in your 2D sketches, viewed with active 3D glasses. Details on how to create a stereoscopic sketch are below.
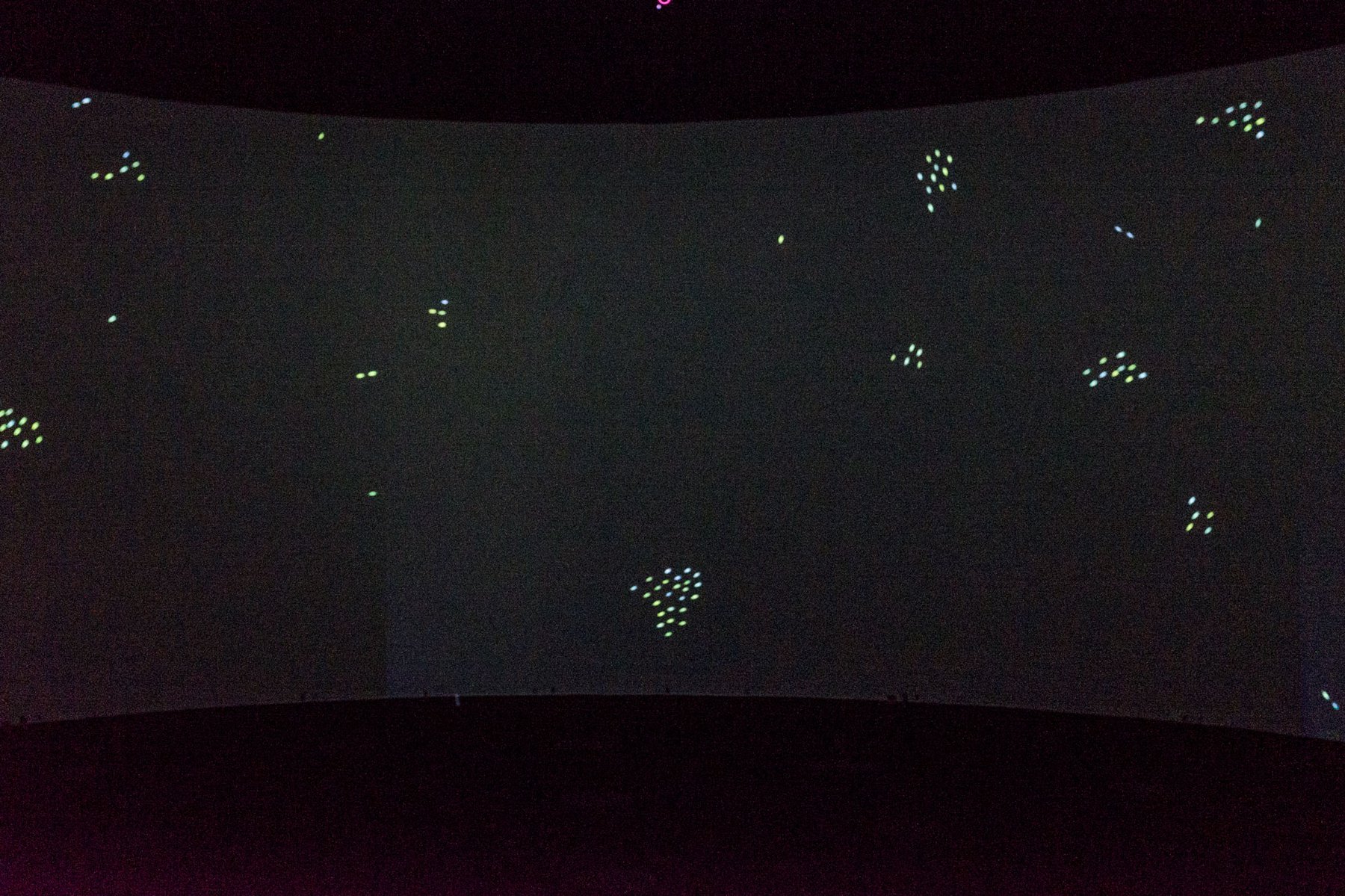
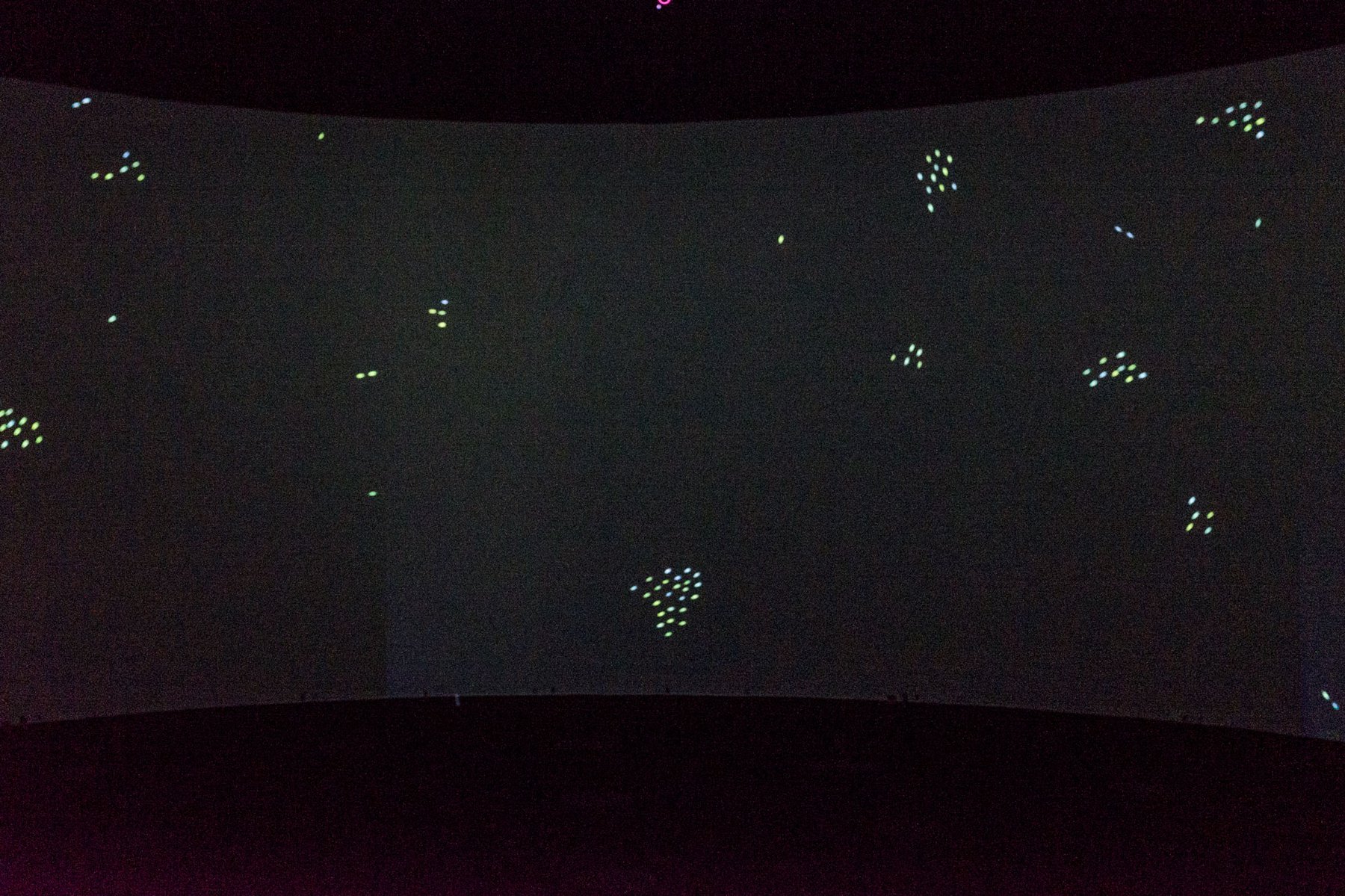
Mono Graphics (standard)
If you’re just interested in mono graphics (like a standard monitor), then you’re good to go. Just make sure your sketch is formatted correctly (see Size and Formatting), and when you’re in the Data Arena, run the following command in a new console window:
matrix.solo.mono
This is an instruction for the video matrix to display mono graphics from Solo (the master computer that Processing runs on in the Data Arena) rather than stereo graphics.
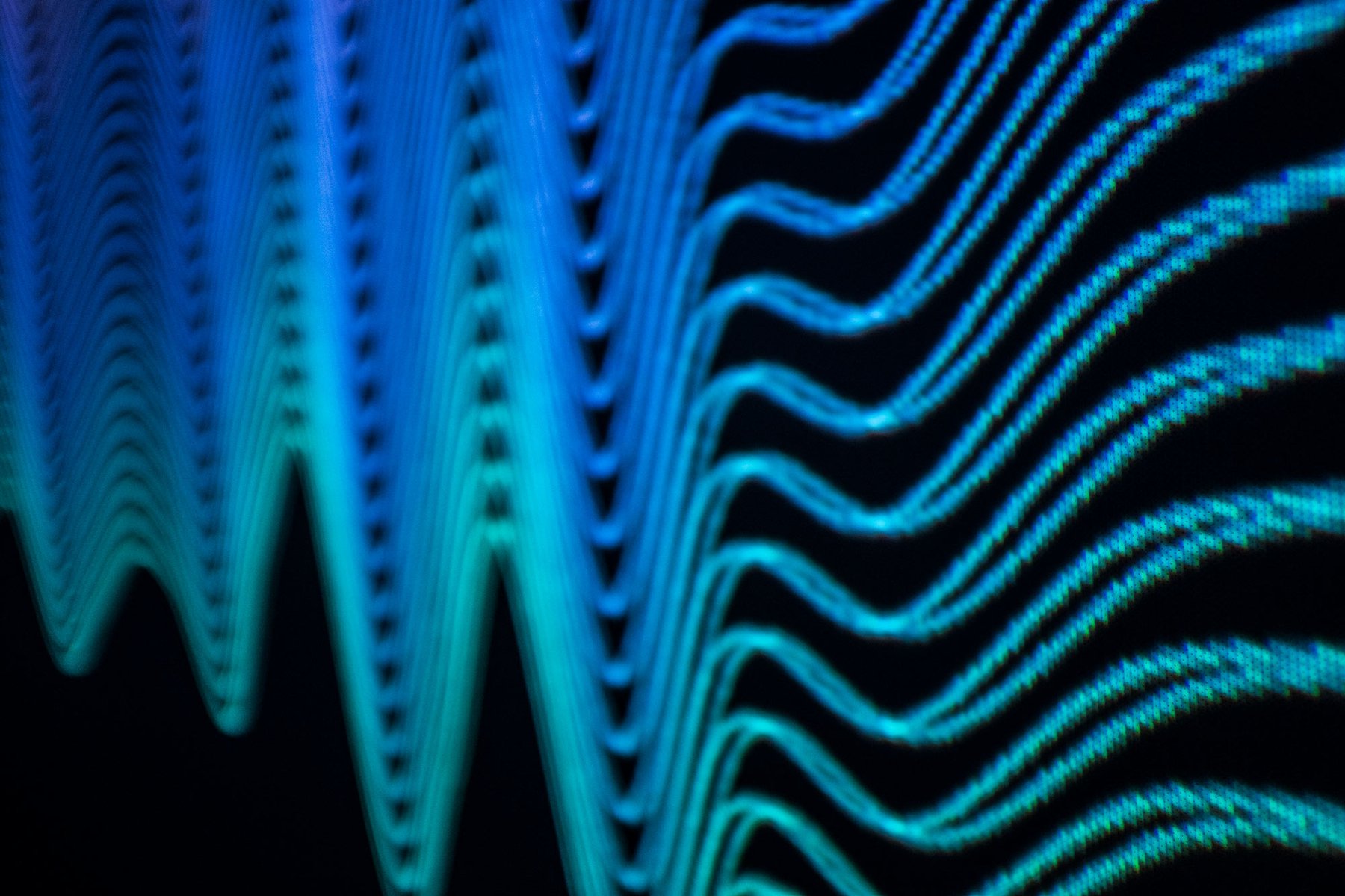
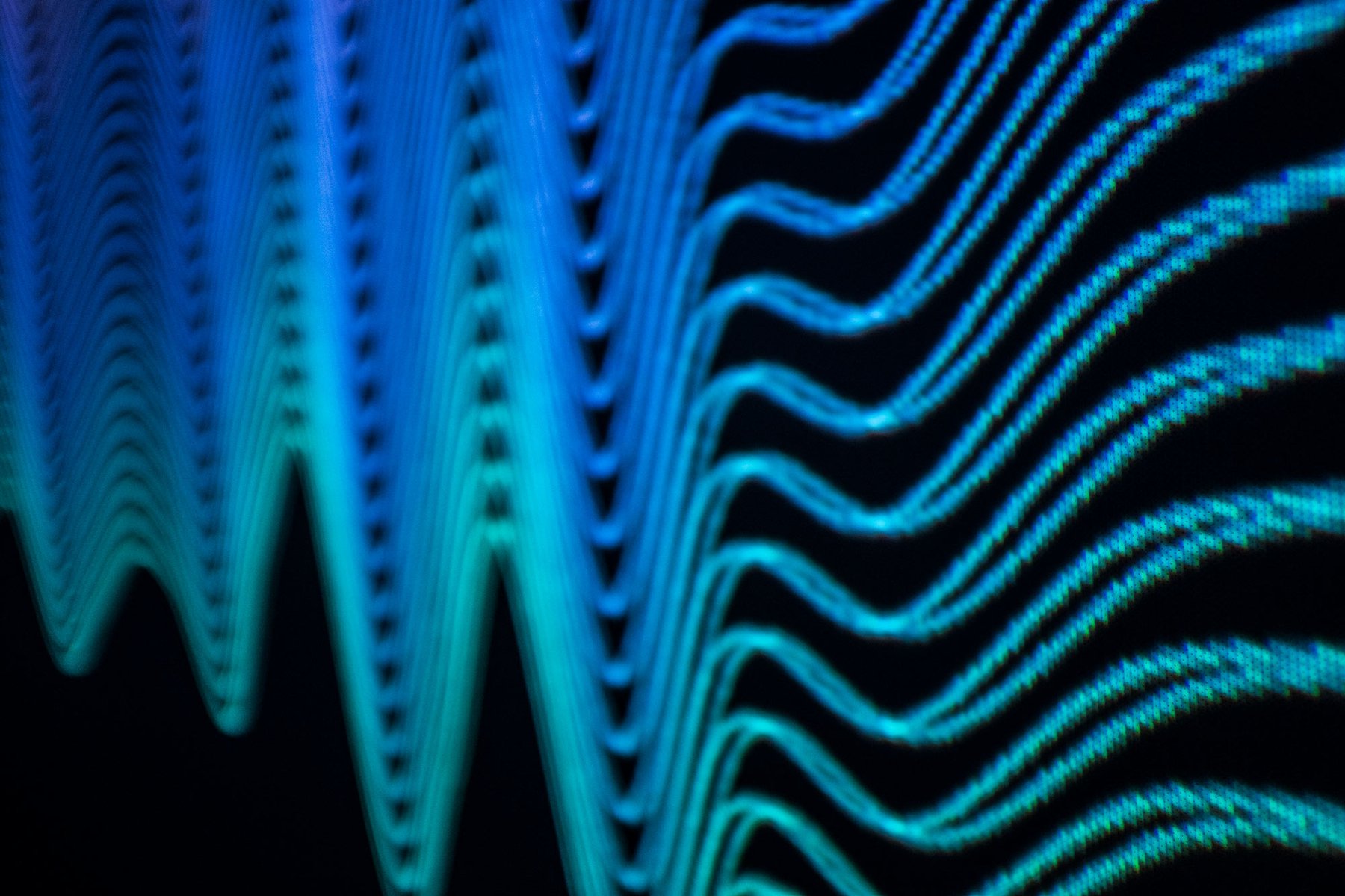
Stereo Graphics
If you’d like to integrate stereoscopic visuals into your 2D sketch, the process is slightly longer. As mentioned above, displaying stereo graphics requires two copies of imagery, stacked vertically (over/under). To help make this clear, have a look at the da_2D_demo_stereoscopy example sketch (can be found here or located in /local/examples/Processing/demos
in the DAVM file system). There is also a stereoscopy template you might like to use to transition your code.
Because two copies of your sketch (stacked vertically, with slight differences) are required, rather than writing twice in the traditional draw()
function, you can use a custom function named drawContents(PGraphics pg, float eyeOffset)
that accepts two arguments: an external PGraphics rendering context, and an offset value. All of your main drawing will happen inside the drawContents()
function, and any Processing functions (e.g fill()
, ellipse()
etc) will be assigned to the PGraphics object pg
:
void drawContents(PGraphics pg, float eyeOffset) {
pg.background(0);
pg.stroke(0, 255, 0);
pg.noFill();
float x = 500;
float y = 300;
float size = 40;
pg.ellipse(x + eyeOffset, y, size, size);
}
You’ll also need to create two PGraphics objects for your top and bottom images. As there will be two images of your sketch in the one document, make sure you set your canvas size to double your intended height, then pass height/2
as the height value for each of your PGraphics objects, so they each become the height of your original sketch (e.g 1200 pixels).
boolean dataArena = true;
PGraphics contentsTop, contentsBottom;
void settings() {
if (dataArena) {
size(displayWidth, 2400, P2D);
} else {
size(1920, 2400, P2D);
}
}
void setup() {
contentsTop = createGraphics(width, height/2, P2D);
contentsBottom = createGraphics(width, height/2, P2D);
// ... all other code for setup
}
This way you only need to write the bulk of your sketch once. Now we can run drawContents()
while drawing to our contentsTop
and contentsBottom
objects in the regular draw()
loop:
void draw() {
contentsTop.beginDraw();
drawContents(contentsTop, 8);
contentsTop.endDraw();
contentsBottom.beginDraw();
drawContents(contentsBottom, -8);
contentsBottom.endDraw();
image(contentsTop, 0, 0);
image(contentsBottom, 0, height/2);
}
When you’re in the Data Arena, run the following command in a new console window:
matrix.solo
This is an instruction for the video matrix to display stereo graphics from Solo (the master computer that Processing runs on in the Data Arena) rather than mono graphics.
Notice the different eyeOffset
values between the top and bottom images. When wearing active 3D glasses, this will cause the ellipse to appear slightly to the left in the left eye, and slightly to the right in the right eye, creating the illusion that it is closer to the viewer (identical to bringing your finger closer to your nose and seeing the image split into two). You’ll have to experiment with this eyeOffset
value to find the right level of depth for your sketch.